Table of Contents
Introduction:
In this article, I am going to explain how to take space-separated input in Python?
There may be a situation when we want to enter more than one value but all the values should be separated by space and we hit the enter key after the last input.
To fulfill this requirement we should know how to take space separated input in Python.
We are using several types of input devices for user input. The standard input device is a keyboard. In this article, I am going to discuss how to take space-separated input in Python using a keyboard.
Program No. 1
x=input().split()
print(x)
After the execution of above code, user can supply space separated input as follows
10 20 30
Output
[’10’, ’20’, ’30’]
Explanation
I am using the input() function to take input from the keyboard. Input() function takes input as a string data type that’s why all the inputs ‘10’ ‘20’ ‘30’ are of type string.
The split() function with zero argument splits the given string on the basis of space and returns a list of elements that’s why we are having the output [’10’, ’20’, ’30’].
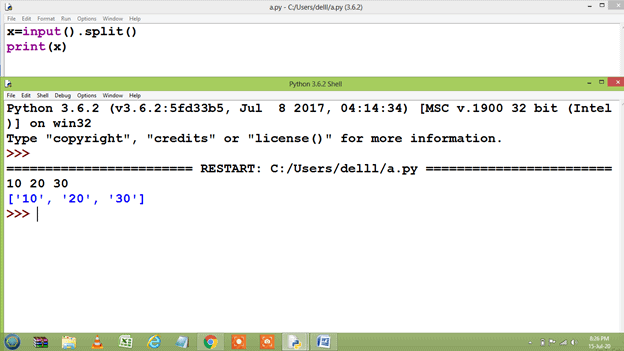
Program No 2
This program is going to show that how to take space separated input in form of integers.
x=list(map(int,input().split()))
print(x)
After the execution of the above code, the user can supply space separated input as follows
10 20 30
Output
[10, 20, 30]
Explanation
I am using the map function to apply the int() function over user input.
map() function is taking two arguments.
The first argument is the name of function I want to apply on user input is the int() function.
Int() function is converting string data type into an integer data type.
I am providing user input as a second argument.
Finally, the list function is converting the object returned by the map function into a list.
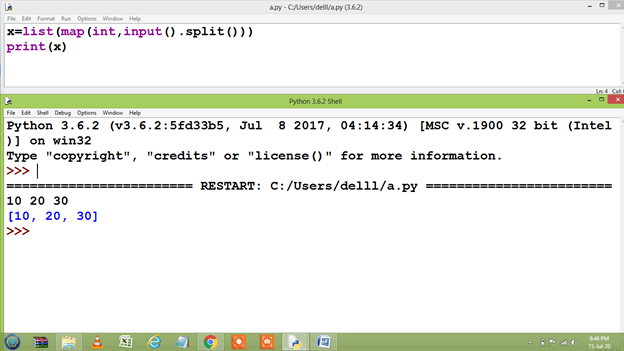
Conclusion
If you are taking input from the keyboard be careful about the data type because by default the data type of the keyboard.