Table of Contents
Fibonacci Series in python
Fibonacci Series in python-In this article, we’re going to start talking about finding the Fibonacci series in python and the factorial of a number in Python.
So to begin with the Fibonacci numbers is a fairly classically studied sequence of natural numbers. The 0th element of the sequence is 0. The first element is 1. And from thereon, each element is the sum of the previous two. So 0 and 1 is 1. 1 + 1 is 2. 1 + 2 is 3. 2 + 3 is 5. And the sequence continues, 8, 13, 21, 34, and so on.
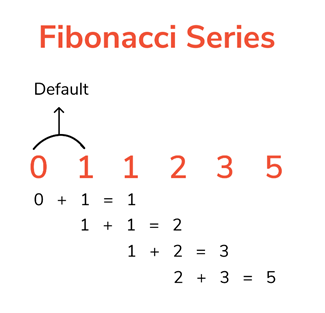
What is Fibonacci series?
Python Program to write Fibonacci Sequence
- First method using Loop
- Second method using Recursion
- Third method using Dynamic Programming
Example of Fibonacci Series: 0,1,1,2,3,5
In the above example, 0 and 1 are the first two terms of the series. These two terms are printed directly. The third term is calculated by adding the first two terms. In this case 0 and 1. So, we get 0+1=1. Hence 1 is printed as the third term. The next term is generated by using the second and third terms and not using the first term. It is done until the number of terms you want or requested by the user. In the above example, we have used five terms.
So let’s take a look at the definition again. The zero’th Fibonacci number is 0. The first Fibonacci number is 1. And from there after each Fibonacci number is the sum of the previous two.
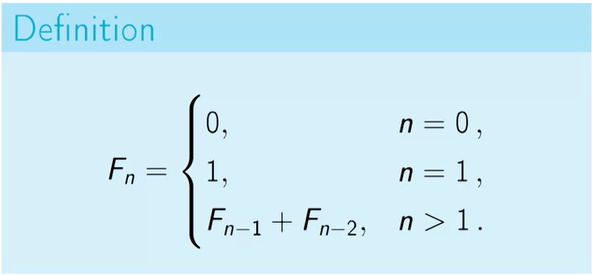
Method 1: Using loop
Python program to print Fibonacci series until ‘n’ value using for loop
# Program to display the Fibonacci sequence up to n-th term
nterms = int(input(“Enter number of terms “))
# first two terms
firstTerm=0 #first element of series
secondTerm=1 #second element of series
if nterms<=0:
print(“Series is “,firstTerm)
else:
print(firstTerm,secondTerm,end=” “)
for i in range(2,nterms):
nextTerm=firstTerm+secondTerm
print(nextTerm,end=” “)
firstTerm=secondTerm
secondTerm=nextTerm
Output:
Enter number of terms 5
0 1 1 2 3
Method 2: Using recursion
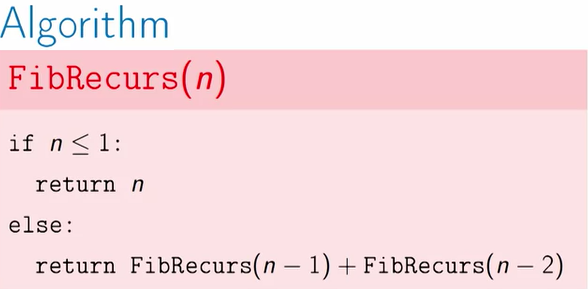
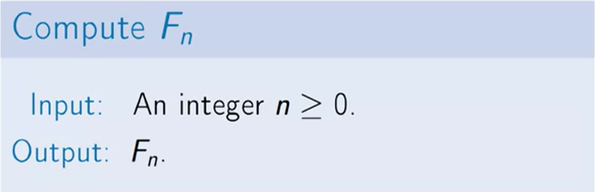
Python program to print Fibonacci series until ‘n’ value using recursion
def fibRecurs(n):
if n<=1:
return n
else:
return fibRecurs(n-1)+fibRecurs(n-2)
# Driver Program
n = int(input(“Enter the value of ‘n’: “))
print(“Fibonacci Series:”, end = ‘ ‘)
for n in range(0, n):
print(fibRecurs(n), end = ‘ ‘)
Output:
Enter the value of ‘n’: 5
Fibonacci Series: 0 1 1 2 3
Python program to print nth Fibonacci number using recursion
def fibRecurs(n):
if n<=1:
return n
else:
return fibRecurs(n-1)+fibRecurs(n-2)
# Driver Program
print(fibRecurs(10))
output:
55
Method 3 (Use Dynamic Programming):
You start off by writing zero and one because those are the first two. The next one going to be zero plus one, which is one. The next one is one plus one which is two, and one plus two, which is three, and two plus three, which is five. And at each step, all I need to do is look at the last two elements of the list and add them together. So, three and five are the last two, I add them together, and I get eight. And, this way, since I have all of the previous numbers written down, I don’t need to do these recursive calls
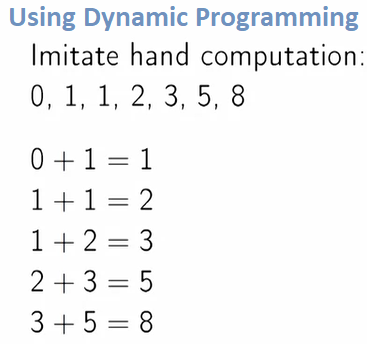
So, let’s see how this algorithm works. What I need to do is I need to create an array in order to store all the numbers in this list that I’m writing down. The zeroth element of the array gets set to zero, the first element gets set to one, that’s to set our initial conditions. Then as i runs from two to n, we need to set the ith element to be the sum of the i minus first and i minus second elements. That correctly computes the ith Fibonacci number.
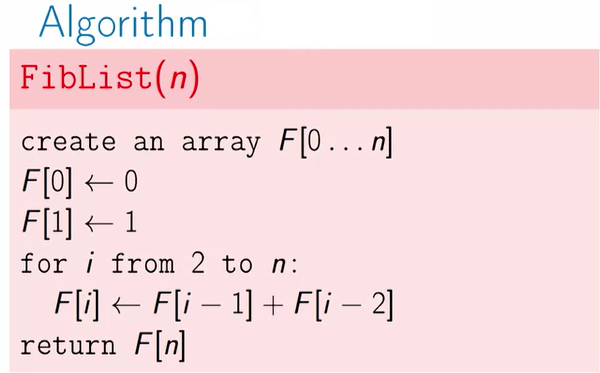
Python program to print nth Fibonacci number using dynamic programming
def fibList(n):
#create an list
fibArray=[0,1]
for i in range(2,n+1):
fibArray.append(fibArray[i-1]+fibArray[i-2])
return fibArray[n]
print(fibList(10))
output:
55
Read More:Python strings |lowercase in python|comparison operators
Python Program to Find the Factorial of a Number
What is a factorial of a number?
Python Program to find factorial of a number
- First Method using Loop.
- Second Method using Recursion.
What is a factorial of a number?
In mathematics, the factorial of a positive integer n, denoted by n!, is the product of all positive integers less than or equal to n: For example, The value of 0! is 1, according to the convention for an empty product.
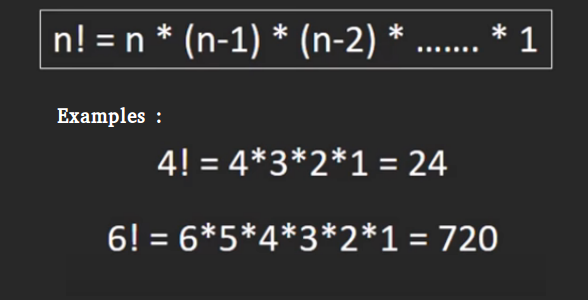
Write an algorithm to find the factorial of a number entered by the user.
First-Step : Start
Second Step : Declare variables n, fact
Third Step: Initialize variables
fact ← 1
Step 4: Read value of n
Step 5: Repeat the steps until n>0
5.1: fact ← fact*n
5.2: n ← n-1
Step 6: Display fact
Step 7: Stop
Method 1(Iterative Method):
Python Program to find factorial of a given number
def factorial(n):
if n<0:
return 0
elif n==0 or n==1:
return 1
else:
f=1
while(n>0):
f=f*n
n=n-1
return f
# Driver Code
num=int(input(‘enter number’))
print(“Factorial of”,num,“is”, factorial(num))
output:
enter number5
Factorial of 5 is 120
Method 2(Recursive Method):
What is recursion?
In simple terms, when a function calls itself it is called a recursion.
Factorial of n
Factorial of any number n is denoted as n! and is equal to
n! = 1 x 2 x 3 x … x (n – 2) x (n – 1) x n
Factorial of 5
5! = 1 x 2 x 3 x 4 x 5
= 120
Factorial Function using recursion
F(n) = 1 when n = 0 or 1
= F(n-1) when n > 1
Step 1: if n==1 then return 1
Step 2: else return n*factorial(n-1)
So, if the value of n is either 0 or 1 then the factorial returned is 1.
If the value of n is greater than 1 then we call the function with (n – 1) value.
def factorial(n):
if n<0:
return 0
elif n==0 or n==1:
return 1
else:
return n*factorial(n-1)
# Driver Code
num=int(input(‘enter number’))
print(“Factorial of”,num,“is”, factorial(num))
output:
enter number5
Factorial of 5 is 120
Method 3(Using Ternary operator):
def factorial(n):
# single line to find factorial
return 1 if (n==0 or n==1) else n*factorial(n-1)
# Driver Code
num=int(input(‘enter number’))
print(“Factorial of”,num,“is”, factorial(num))
output:
enter number 5
Factorial of 5 is 120
Read the more interesting article: click here
Read the more interesting article: Database Developer Skills and Salary